Blog
July 12, 2019Combining SwiftUI with ViewControllers
By now, everyone has heard of SwiftUI and knows that it is a new toolkit that Apple has introduced. SwiftUI lets us design an app's UI in a declarative way. Simply give SwiftUI a set of rules and it figures out how to display the content.
When I first started playing around with SwiftUI, I got really involved in building out separate views and then combining them together into one complex view. The ability to see your changes instantly as you build out your view on a live simulator or device is a nice new improvement tool. This new toolkit is very powerful. In time, SwiftUI may replace UIKIt but for now, UIKit is here to stay. SwiftUI requires iOS 13 and there are a lot of users that don’t update to the latest version of iOS when it is first released. In order to develop with SwiftUI full capabilities, you must have XCode 11 and run it on Mac 10.15 (Catalina).
There are a couple of ways that you can combine UIKit ViewControllers and SwiftUI views. Let's say you have a ViewController that has your built out functionality but you want to add it to your SwiftUI view. You could make your ViewController conform to the UIViewControllerRepresntable protocol. This protocol has 2 functions, makeUIViewController and updateUIViewController that must be implemented.
Instead of making each of your UIKit ViewControllers conform to the UIViewControllerRepresentable protocol, you could simply make a wrapper and tell the wrapper which ViewController you want to use inside of the SwiftUI view. Below is an example that I called ViewControllerWrapper.
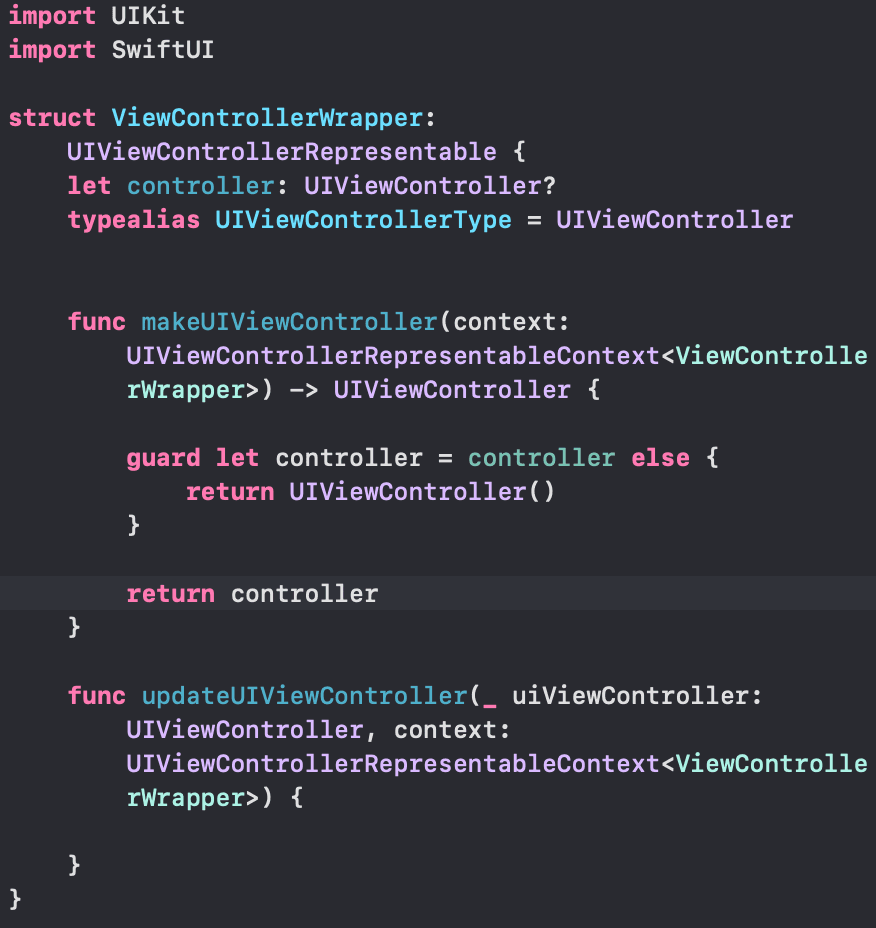
Inside of your SwiftUI view, you would just call this wrapper and pass it to the UIKit ViewController that you want to use. You can also manipulate the view inside of updateUIViewcontroller. Apple provides a tutorial on this functionality. https://developer.apple.com/tutorials/swiftui/creating-and-combining-views
Placing a SwiftUI view inside of a viewController
Let’s say you have a custom SwiftUI view that you want to incorporate into your existing viewController. You can add the SwiftUI view by importing SwiftUI framework and then bring it in by using UIHostingController(rootView: someView()). You can then grab the view and add it to your viewController the same way that you add any UIKit view. I have provided an example below.
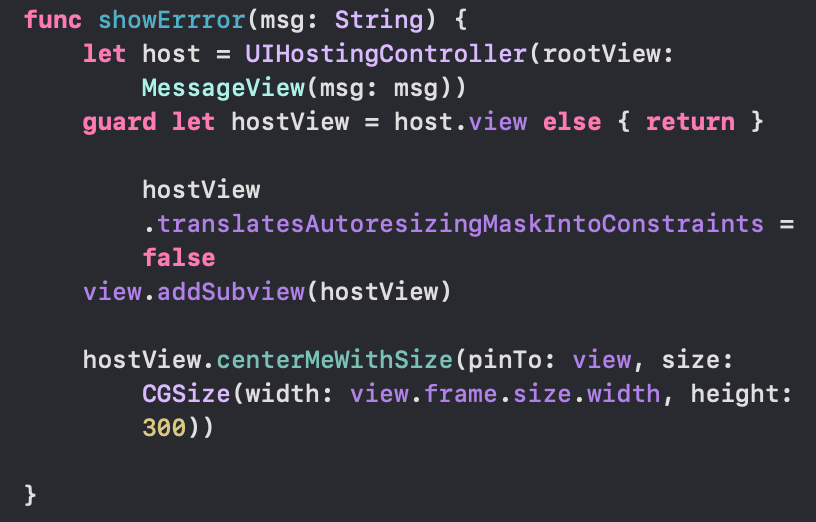
Note: If you do decide to keep your existing viewControllers and mix with the new SwiftUI views, be careful in how you design your code because you are not able to directly update SwiftUI declarative values inside of a viewController.
SwiftUI is still relatively new but it is a powerful framework that allows you to code visually. Even though UIKit is not going anywhere anytime soon, I encourage you to get to know SwiftUI inside and out in order to be ready for future iOS developments.