The classic findViewById method is as old as Android itself, yet remains the most common approach to accessing views. Over the years, flashy new methodologies have threatened to usurp it, but none succeeded. Butterknife has its charms; Data Binding is feature-rich but clunky to implement. Kotlin synthetic is its most worthy challenger, but lacks compile-time safety and doesn't work in Java.
Perhaps some developers enjoy entering findViewById over and over again. (It builds character! In my day, we had to cast the view! Quit your bellyachin'!) But for the rest of us, we've been waiting for a comprehensive solution to this problem for some time.
Buried in an IO talk about Architecture Components, Google presented a new view accessor methodology that, at long last, may finally topple findViewById once and for all: View Binding.
The Problem
Yigit Boyar concisely defined the low-key headache every Android developer has faced for years when he stepped through this chart:
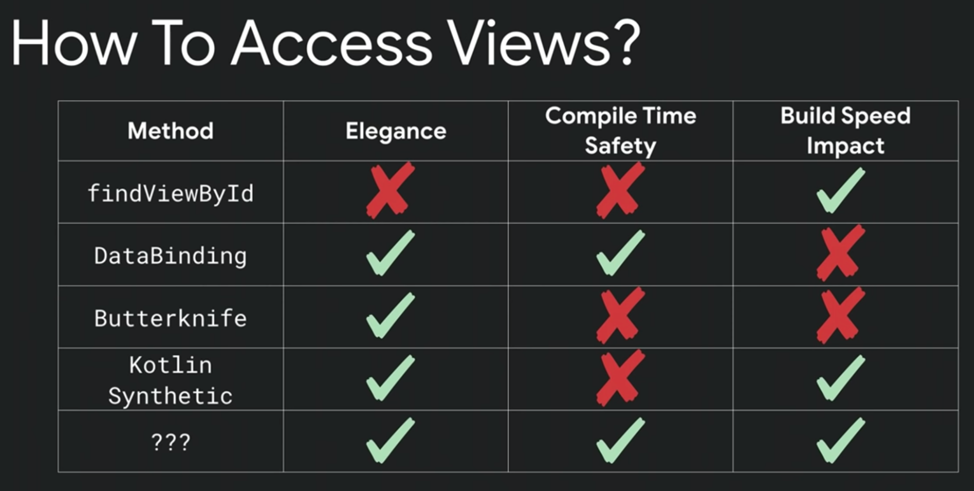
Each option has flaws:
- FindViewById: Our never-fail clunker that's gotten us through hard times, but threatens to give us carpal tunnel.
- Data Binding: A library too powerful for its own good. Its annotation processing causes sluggish build times as IntelliJ churns through layout after layout.
- Butterknife: A popular third-party library by Android legend Jake Wharton. It also suffers from APT slowdowns, and peaked in popularity back when views required casting.
- Kotlin Synthetic: The best option so far, but still suffers from compile-time safety issues and only supports Kotlin.
Google acknowledges that many developers use Data Binding solely for the views — without any additional logic or object references. Most seasoned Android developers believe strongly in the separation of concerns, and structure their codebases in MVP or MVVM to satisfy this tenet. As a result, Google's Data Binding, and its ability to add logic inside the XML directly, struck many developers as somewhere between counterintuitive and sacrilegious. We don't want to execute logic in the XML file — we just want the views bound automatically without entering line after line of boilerplate code.
The Solution
Introduce View Binding, slated for release in Android Studio 3.6. View Binding keeps things simple, only concerning itself with binding your views to your fragment/activity. It requires no modification of your XML files, utilizes a dead-simple implementation, and satisfies all three of our functional criteria: it's elegant, compile-time safe, and causes minimal build speed impact. (It also works for Java.)
We'll borrow Yigit's example from IO:
<!-- profile.xml -->
<LinearLayout>
<TextView android:id="@id/title" />
<ImageView android:id="@id/photo" />
</LinearLayout>
class ProfileActivity: AppCompatActivity {
override fun onCreate(savedInstanceState: Bundle?) {
val binding = ProfileBinding.inflate(layoutInflator)
setContentView(binding.root)
// binding.title : TextView
// binding.photo: ImageView
}
}
- Elegance: Our code can't get much less verbose than this.
- Compile-Time Safety: Inflating with ProfileBinding guarantees your bindings are 100% safe. No more inflating and just hoping your views will be there when you expect them to be.
- Quick Builds: All binding classes are generated by the Android Gradle plugin. Layout bindings are only rebuilt when modified, and are done so incrementally.
This approach comes with full Android Studio integration and supports interoperability with Data Binding. If you've been using Data Binding solely to bind your views, you can simply replace Data Binding with View Binding and your code will compile successfully (without all the slowdowns).
Conclusion
Though certainly less heralded than other IO announcements, View Binding offers a marginal improvement to a rote task Android developers perform on a day-to-day basis. This showcases Google's willingness to continue improving the mundane aspects of Android development, instead of focusing entirely on their platform's showier aspects.
View Binding is slated to roll out with Android Studio 3.6. Will findViewById survive us all, or are its days finally numbered?